Fluid Player Master Class #2: How to add ad zones
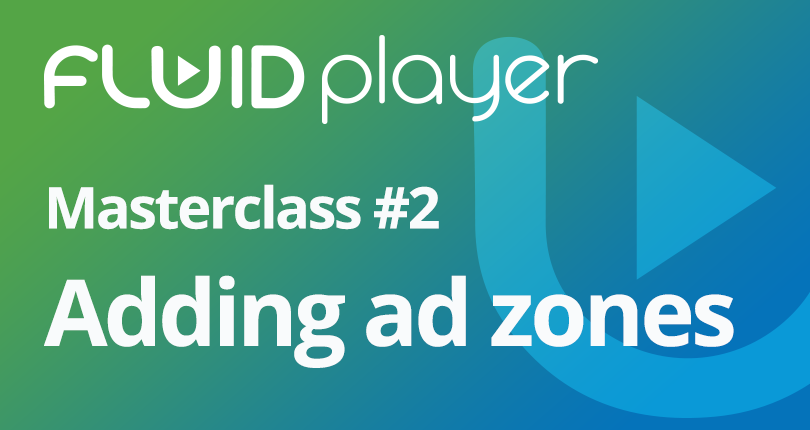
If you are not an expert in writing code, this Master Class will show you the code you need to add different ad zones using VAST, so you can easily add it to your website to monetise your video content through Fluid Player.
The In-Video (VAST) format allows publishers to have one or multiple ads displayed along a video’s timeline within Fluid Player.
Here is a full list of the JavaScript code parameters:
- adList: This will allow you to set up one or multiple VastTags. (Please note the VAST tag XML response Content-Type must be either application/xml or text/xml).
- roll (mandatory): This is used to set the timeline position of Pre-Roll, Mid-Roll, OnPause, Post-Roll.
- vastTag (mandatory): Ensure that you set it with the url of the VAST XML (Please find the supported tags/attributes here vastLinear.xml).
- timer (only for mid-roll): This schedules when the ad(s) play either after the specified number of seconds or after a certain percentage of the video content has been viewed.
- nonLinearDuration (only for nonLinear, optional): The number of seconds until the nonLinear Ad will be shown. If this is not set, the in-video ad will be displayed until the user clicks close [x] or the ad will be displayed until the video ends.
Resources
The following code is where the Fluid Player resources are placed:
First, add this code within the <head> tag: <!-- Style --> <link rel="stylesheet" href="https://cdn.fluidplayer.com/v2/current/fluidplayer.min.css" type="text/css"/> <!-- Main Fluid Player Core code --> <script src="https://cdn.fluidplayer.com/v2/current/fluidplayer.min.js"></script> <!-- Style --> <link rel="stylesheet" href="https://cdn.fluidplayer.com/v2/current/fluidplayer.min.css" type="text/css"/> <!-- Main Fluid Player Core code --> <script src="https://cdn.fluidplayer.com/v2/current/fluidplayer.min.js"></script> Then add this code within the <body> tag //Video content <video id="video-ad-list" controls> <source src="https://cdn.fluidplayer.com/videos/valerian-720p.mkv" title="720p" type="video/mp4"> </video>
Ad Format code
Each of the codes below define the different options you have to add video ads into the player. This code should be added after the </video> tag.
Pre-Roll
This is the video ad list including the pre-Roll VAST file.
<script type="text/javascript"> var testVideo = fluidPlayer( 'video-ad-list', { vastOptions: { "adList" : [{ "valign" : "bottom", //only for In-Video "roll" : "preRoll", "vastTag" : "https://adservingdomain.com/splash.php?idzone=0000000" }] } } ); </script>
Mid-Roll
This is the video ad list that includes the Mid-Roll custom options. You can have multiple Mid-Rolls however, only one Pre-Roll and one Post-Roll can be set. Below are two pieces of code, the only difference between them is the way the timer parameter is selected. You can choose the number of seconds “timer” : “8” which will show the ad 8 seconds into the video or you can choose the percentage of video that is show before the ad is shown “timer” : “25%”
<script type="text/javascript"> var testVideo = fluidPlayer( 'video-ad-list', { vastOptions: { "adList" : [{ "valign" : "top", //only for In-Video "roll" : "midRoll", "timer" : "8", "nonlinearDuration" : "5", //only for In-Video "vastTag" : "https://adservingdomain.com/splash.php?idzone=0000000" }, { "valign" : "middle", //only for In-Video "roll" : "midRoll", "timer" : "25%", "nonlinearDuration" : "5", //only for In-Video "vastTag" : "https://adservingdomain.com/splash.php?idzone=0000000" }, { "roll" : "midRoll", // In-stream ad "timer" : "75%", "vastTag" : "https://adservingdomain.com/splash.php?idzone=0000000" }] } } ); </script>
On-Pause
This is the video ad list that includes the On-Pause option.
<script type="text/javascript"> var testVideo = fluidPlayer( 'video-ad-list', { vastOptions: { "adList" : [{ "valign" : "bottom", "roll" : "onPauseRoll", "vastTag" : "https://adservingdomain.com/splash.php?idzone=0000000" }] } } ); </script>
Post-Roll
This is the video ad list that includes the Post-Roll option.
<script type="text/javascript"> var testVideo = fluidPlayer( 'video-ad-list', { vastOptions: { "adList" : [{ "valign" : "top", //only for In-Video "roll" : "postRoll", "vastTag" : "https://adservingdomain.com/splash.php?idzone=0000000" }] } } ); </script>
Using all of the ad formats together
Here is the full list of all the video ad options above grouped as one piece of code:
<script type="text/javascript"> var testVideo = fluidPlayer( 'video-ad-list', { vastOptions: { "adList" : [ //preRoll { "vAlign" : "bottom", //only for In-Video "roll" : "preRoll", "vastTag" :"https://adservingdomain.com/splash.php?idzone=0000000" }, //midRoll { "valign" : "top", //only for In-Video "roll" : "midRoll", "timer" : "8", "nonlinearDuration" : "5", //only for In-Video "vastTag" : "https://adservingdomain.com/splash.php?idzone=0000000" }, { "roll" : "midRoll", // In-sream ad "timer" : "75%", "vastTag" : "https://adservingdomain.com/splash.php?idzone=0000000" }, //onPauseRoll { "valign" : "bottom", //only for In-Video "roll" : "onPauseRoll", "vastTag" : "https://adservingdomain.com/splash.php?idzone=0000000" }, //postRoll { "valign" : "top", //only for In-Video "roll" : "postRoll", "vastTag" : "https://adservingdomain.com/splash.php?idzone=0000000" } ] } } ); </script>
In this final example this is how the complete code should look for the insertion of one Pre-Roll ad:
<html> <head> <!-- Style --> <link rel="stylesheet" href="https://cdn.fluidplayer.com/v2/current/fluidplayer.min.css" type="text/css"/> <!-- Main Fluid Player Core code --> <script src="https://cdn.fluidplayer.com/v2/current/fluidplayer.min.js"></script> </head> <body> <!-- Video content --> <video id="video-ad-list" controls> <source src="https://cdn.fluidplayer.com/videos/valerian-720p.mkv" title="720p" type="video/mp4"> </video> <script type="text/javascript"> var testVideo = fluidPlayer( 'video-ad-list', { vastOptions: { "adList" : [ //preRoll { "vAlign" : "bottom", //only for In-Video "roll" : "preRoll", "vastTag" :"https://adservingdomain.com/splash.php?idzone=2947418" } ] } } ); </script> </body> </html>
For more information please visit Fluid Player’s documentation page.
Fluid Player Community
Fluid Player is open source, so join the community and see what new features developers are creating for Fluid Player on GitHub.